A string is a sequence of characters, such as letters, numbers, punctuation marks and/or special characters. They are referred to as alphanumerics (alphabet characters and/or numbers).
The String Class is provided by Java
to allow
for the discussion and use of String variables. In Java, strings are objects, not
primitive data types such as int, double, char, and boolean.
As such, you must capitalize String since it is the name of a class.
The term string literal refers to any alphanumeric (character, digit, or both) enclosed in
double quotation marks (such as "Snoopy" or "123 West Third
Street").
If a string literal contains only
numeric digits ("1234"), it is NOT a number
(an integer). Be careful! It
is just a string of numeric digits that cannot be used to
perform mathematical calculations such as addition or
multiplication. Remember that you can perform
mathematical calculations on numeric data only, not on string literals.
"1234" is not the same
as 1234
"1234" is a string
1234 is a number |
|

Declaring a String variable is
different from declaring an int, double, char or boolean. The variable name
of a String points to a String object that is allocated in memory (the actual location where the
string is stored).
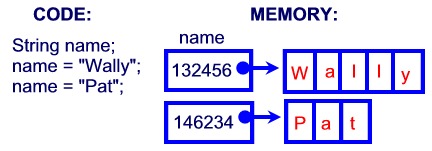
Each time a new assignment is stored in the String variable "name", the computer
finds a new memory location to hold the String text and adjusts the contents of "name" to
hold the address of this new location. The old memory location
is set free.
Variables used in this manner (such as "name")
are called references. The address values stored in
these variables are said to "point" to the actual data.
The string object in memory is made up of individual characters (chars) as shown below. These characters are indexed starting with the number zero.
Notice that the
index of the last char is one less than the actual length of the string.

These indexes will be used to access "parts" of the string variable, as we will see in the String Methods section at the bottom of this page.
If you assign a null to a string, use the null keyword. The String variable will not point to a String object that's allocated in memory. String variable = null;
Do not confuse the null string with an empty string. String variable = "";
The empty string is coded with a set of quotation marks with no space between them. This String variable value is known, but the string does not contain any characters.
Examples of declaring and initializing strings:
String greeting; // declare the string
greeting = "Hello"; //initialize the string
String title = "Captain"; //declare
and initialize at one time
System.out.println(title); //print
String poem = reply.nextLine();
//This code reads all characters a user types up to the pressing of the Enter key.
//This input process will be examined further in the next lesson. |

Printing String variables: The statement below was seen in the Printing section. We now know that the "literal print" in this statement is in fact a "string literal".
System.out.println(" The total pay is " + totalPay);
The + (plus) operator that we saw at work in the statement above is part of a process referred to as concatenation. Concatenation is the combining or merging of two String fields using the + operator. In the statement above, the "+" sign concatenates the string literal with the value that is
stored in the variable totalPay. While totalPay is declared as a double in the program, it is automatically converted to a String for
the purpose of concatenation and the subsequent print out.
Example:
String firstName = "Darth";
String lastName = "Vader";
You can use concatenation to store a full name:
String fullName = firstName + " " + lastName;
Or you can use concatenation to simply print the full name:
System.out.println(firstName + " " + lastName);
Prints to screen: Darth Vader
Notice the need of the " " (blank space) to separate the first name from the last name.
Potential problem concatenating
Strings with numerics:
System.out.println("answer " + 3 + 4); // becomes answer 34
System.out.println("answer " + (3 + 4)); // becomes answer 7
As suggested in the Printing section, placing numeric values in parentheses will help avoid this order of operations problem with the "+".
The concatenation operator (+) has the same precedence as addition,
which may occasionally cause strange results.
|

String Methods:
String is a "class" and as such has methods that are properties of the class.
To use a method, we need the dot operator.
- length( ) This is a
numerical returning method that gives the length
of the string. It returns an integer value.
Example:
String city = "Fulton";
int citylength = city.length(); //stores the length of city into an int
System.out.println(citylength); //prints the
length of the string which is 6
- charAt(n)
This is a value returning method. The argument states which character to return. The
subscripting of the locations of the characters starts with zero.
Example:
String holiday="Thanksgiving";
System.out.println(holiday.charAt(4)); //prints out
a 'k'
Example:
String puppy ="Wally";
System.out.println(puppy.charAt(0)); //prints out a 'W'
- trim( ) This is a
value returning method that returns a copy of the
argument after removing its leading and trailing blanks (not embedded blanks -
blanks within the statement). The string will not be changed in
memory.
Example:
String burp = " hic
up ";
System.out.println(burp.trim()); // prints out hic up
- toLowerCase( )
In memory, the string is not changed, but it will be printed out in all lower
case letters.
Example:
String cityname = "Houston";
System.out.println(cityname.toLowerCase()); // prints out houston
- substring(Start, End)
This is a value returning method. A string is returned beginning at Start
subscript up to but not including the End subscript.
Example:
String sport = "football";
System.out.println(sport.substring (1,3)); // prints out oo
substring(Start)
This is an alternate version. It returns the substring beginning at Start
subscript up to and including the end of the string.
Example:
String sport = "football";
System.out.println(sport.substring(1)); // prints out ootball
- indexOf("Garfield") Returns the beginning subscript of the string where
"Garfield" is found
Example: (find the comma in a city, state zip listing)
String city = "Fulton, New York 13069";
System.out.println (city.indexOf (",")); // prints 6
- equals( ) Used
to compare two strings with the method to see
if they are exactly the same, this includes any blanks or spaces within the
string.
Example: (check to see if the
user entered a dog name of Snoopy)
if (dogname.equals("Snoopy"))
System.out.println("The user entered Snoopy.");
- compareTo( ) Compares strings to determine alphabetic location. Returns a zero if the two strings are equal,
a negative if the first string is alphabetically before the compared string,
and a positive if the first string is alphabetically after the compared
string.
Example:
String subject = "mathematics";
boolean answer;
answer = subject.compareTo ("biology");
// answer is positive - math comes after biology
answer = subject.compareTo("philosophy");
//answer is negative - math comes before philosophy
answer = subject.compareTo ("mathematics");
//answer is zero - math is math
|
|