Problems with getline(cin,
name); |
Since getline
does not ignore leading
whitespace characters, you should take special care when using it in
conjunction with cin >>.
The
problem: cin>>
leaves the newline character (\n) in the iostream. If getline
is used after cin>>,
the getline
sees this newline character as leading whitespace, thinks it is finished
and stops reading any further.
Program
fragment with problem:
#include
"apstring.cpp"
apstring name;
int age;
cout<<"Enter your
age";
cin>>age;
cout<<"Enter your full name";
getline(cin, name);
cout<<name<<",
you are "
<<age<<endl;
|
OUTPUT:
Enter your age 5
Enter your full name Ben Bunny
, you are 5
The
name did not print because the getline saw the newline character
left from the cin>> as whitespace and stopped reading any
further. |
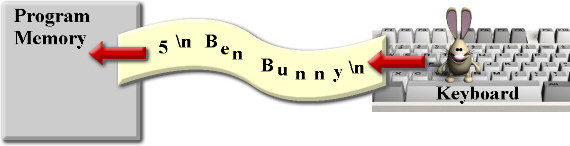
The yellow wave is the iostream.
The cin statement uses the 5 and leaves the \n in the stream
as garbage. The cin statement will NOT read (or
"grab") \n. The cin also ignores \n when
reading data. The getline, on the other hand, reads and
"grabs" \n. So, when it sees the \n
LEFT from cin, it "grabs" the \n and
thinks it is finished reading.
Don't
be a dumb bunny and fall for this trap!!!
Ways to fix the problem:
Method
1:
Reverse the order of the input.
Avoid putting getline after cin
>>.
This
method has limited applications. If you are looping, this
will not solve the problem. |
Method
2:
Consume the trailing newline
character from the cin>> before
calling getline, by
"grabbing" it and putting it into a "dummy"
variable.
You
will see me using this method throughout the course.
apstring dummy;
getline(cin, dummy);
or
cin.ignore(100,'\n');
//
skips up to 100 characters
// stopping after it finds and "grabs" \n |
Program fragment with
correction:
#include
"apstring.cpp"
apstring name, dummy;
int age;
cout<<"Enter your
age";
cin>>age;
getline(cin,dummy);
cout<<"Enter your full name";
getline(cin, name);
cout<<name<<",
you are "
<<age<<endl;
|
OUTPUT:
Enter your age 5
Enter your full name Ben Bunny
Ben Bunny, you are 5
|
|