Sorting an array of structures is basically the same as sorting any other array.
The only difference is the comparison statement.
In an array of integers, the array is subscripted to perform the comparison.
The statement which then checks to see if the array elements are in the proper order
might look like:
if ( numbers[ i ] > numbers[ j ] )
// exchange sort
{ . . .
}
|
Unfortunately, this will not be sufficient for an array of structures.
Such a comparison would try to compare entire structures to one another, rather than comparing members of the structure.
When working with structures, you must be sure that the
comparison statement is operating on the correct member of the structure.
This example will send an array of structures to a function to be sorted.
//Sorting
Structures
//Information on brands of printers in stock
#include <iostream.h>
#include <apvector.h>
#include "apstring.cpp"struct PRINTER_TYPE
{
apstring brand;
int modelNumber;
double cost;
} ; |
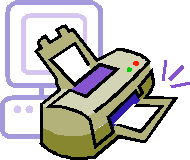 |
void sort(apvector <PRINTER_TYPE> &printerStock);
int main(void)
{
apvector <PRINTER_TYPE> printerStock(30);
. . .
sort (printerStock);
. . .
}
// function to sort array of structures . . . abc order by
brand
void sort (apvector <PRINTER_TYPE> &printerStock)
{
PRINTER_TYPE temp;
for(int i = 0; i < printerStock.length( ) - 1; i++)
{
for (int j = i + 1; j <
printerStock.length( ); j++)
{
if (printerStock[ i ].brand > printerStock[ j ].brand) //comparing
brands
{
temp = printerStock[ i ]; //swapping entire
struct
printerStock[ i ] = printerStock[ j ];
printerStock[ j ] = temp;
}
}
}
return;
}
|