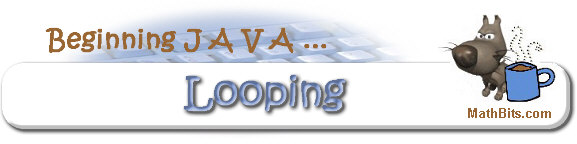
Return to Unit
Menu | Java Main Page |
MathBits.com |
Terms
of Use
The Math Class
While the Math class is quite extensive, we will limit our attention to
the
methods listed below for the time being.
Method |
Purpose |
abs(int x) |
Returns the absolute value of an integer x. |
abs(double x) |
Returns the absolute value of a double x |
pow(double base, double
exponent) |
Returns the base raised to the exponent. |
round(double x) |
Returns x rounded to the nearest whole number.
Returned value must be cast to an int before assignment to an int
variable. |
max(int a, int b) |
Returns the greater of a and b. |
min(int a, int b) |
Returns the lesser of a and b. |
double sqrt(double x) |
Returns the square root of x. |
random( ) |
Returns a random number greater than or equal to 0.0
and less than 1.0. |
These methods are used by invoking the name of the
class (Math), followed by a dot (.), followed by the name of the method
and any needed parameters.
SYNTAX:
radius = Math.sqrt(area/Math.PI));
More Examples:
int ans1;
double ans2;
ans1 = Math.abs(-4);
//ans1 equals 4
ans1 = Math.abs(-3.6);
//ans1 equals 3.6
ans2 = Math.pow(2.0,3.0);
//ans2 equals 8.0
ans2 = Math.pow(25.0, 0.5); //ans2 equals 5.0
ans1 = Math.max(30,50);
//ans1 equals 50
ans1 = Math.min(30,50);
//ans1 equals 30
ans1 = (int) Math.round(5.66); //ans1
equals 6
Random Number Generation
Computers can be used to simulate the
generation of random numbers with the use of the
random( )
method. This random
generation is referred to as a pseudo-random generation. These created
values are not truly "random" because a seeded mathematical formula is
used to generate the values.
Remember that the
random( ) method will
return a value greater than or equal to zero and less than 1. But
don't expect values necessarily like .5 or .25. The following line
of code:
double randomNumber = Math.random( );
will most likely generate a
number such as 0.7342789374658514. Yeek!
When you think of random numbers for a game or an
activity, you are probably thinking of random "integers". Let's do
a little adjusting to the random(
) method to see if we
can create random integers.
Generating random
integers within a specified range:
In order to produce random
"integer" values
within a specified range, you need to manipulate the random( ) method.
Obviously, we will need to cast our value to force it to become an
integer.
The formula is:
int
number = (int) (a + Math.random( ) * (b - a + 1));
a
= the first number in your range
b
= the last number in your range
(b - a + 1 )
is the number of terms in your
range.
(range computed by largest value - smallest value + 1)
Examples:
• random integers in the interval from 1 to 10 inclusive.
int number = (int) (1 + Math.random( ) * 10);
• random integers in
the interval from 22 to 54 inclusive.
int number = (int) (1 + Math.random( ) * 33);
note: the number of
possible integers from 22 to 54 inclusive is 33
b - a + 1 = 54 - 22 + 1 = 33

Return to Unit
Menu | Java Main Page |
MathBits.com |
Terms
of Use
|