. |

Return to Unit
Menu | Java Main Page |
MathBits.com |
Terms
of Use
What is Actually Passed to a
Method?
In Java, parameters sent to methods are
passed-by-value:
Definition clarification: What is passed
"to" a method is referred to as an "argument". The "type"
of data that a method can receive is referred to as a "parameter".
(You may see "arguments" referred to as "actual
parameters" and "parameters" referred to as "formal parameters".)
|
First, notice that if the arguments
are variable names, the formal parameters need not be
those same names (but must be the same data type).
Pass-by-value
means that when a method is called, a copy
of the value of each argument is passed to the
method. This copy can be changed
inside the method, but such a change will have
NO effect on the argument. |
Let's look at some examples:
Ex. 1:
Will the values from main be changed after being sent to this
method?? |
int num = 10;
double decimal = 5.4;
NumberManeuvers(num, decimal);
System.out.println("num = " + num + "and
decimal = " + decimal);
----------------------------------------------------------------
public static void NumberManeuvers(int i, double j)
{
if (i == 10)
j = 6.2;
i = 12;
}
// ANSWER: In this case, NO.
The output will be:
num = 10 and decimal = 5.4
|
Here's what's happening!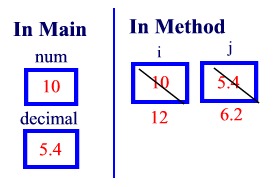
Copies of the actual parameter values from main are sent
to the method, where they become the values for the formal
parameters. When the method is finished, the copies are
discarded. The actual parameter values remain unchanged.
(Notice that nothing was "returned" from this method.) |
Ex. 2:
What will happen to the values in main after being sent to this
method?? |
int a =
2, b = 3, c = 4, answer = 2;
answer = discriminant(a, b, c);
System.out.println("The discriminant is " + answer);
------------------------------------------------------------
public static int discriminant(int x, int y, int z)
{
int disc;
disc = y*y - 4*x*z;
return disc;
} |
Here's what's happening!
It is possible for a method to change a value in main by
returning and saving the value after the method is complete.
The limitation to this concept is that only ONE value can
be returned from a method.
In this example, answer was initially 2, but after execution of
the method and new assignment to answer, it now has the value
-23. |
Ex. 3:
Will the happen to the String dog after dog is sent to this
method?? |
String
dog = "My dog has fleas."
dog = SpeciesChange(dog);
System.out.println("dog);
public static String SpeciesChange(String pet)
{
pet = "My cat has fleas.";
return (pet);
} |
Here's what's happening!
As in the examples above, since the method returns a value that
is then stored in dog, the value of
dog
is changed. The output is:
My cat has fleas.
If no value had been
returned, there would have been no change to
dog. |

Return to Unit
Menu | Java Main Page |
MathBits.com |
Terms
of Use
|