|
Built-In Routines for Arrays |
The Arrays class (java.util.Arrays) is a set of built-in static methods that are useful when working with arrays. The Arrays class contains built-in methods for searching and sorting, as well as a variety of other methods.
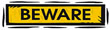 There are often built-in sort "routines"
in Java compiler packages,
such as Arrays.sort ( ).
It is imperative that you, the programmer, know and understand at least one
method of sorting
an array, separate from a built in "routine", for
test taking purposes. |
• sort (array) - sorts an array into ascending order |
Example - Using Arrays.sort( )
//
short demo to show built-in sorting routine at work
import java.util.Arrays; //notice the new required import statement
public class SortTest
{
public static void main(String[] args)
{
//Sorting integers
int[ ] nums = {12, 18, 7, 20, 11};
Arrays.sort(nums); //use built-in sorting method
for (int i = 0; i < 5; i++)
System.out.println(nums[ i ]);
//Sorting strings
String[ ] people = {"Wally","Henry","Felix","George"};
Arrays.sort(people); //use
built-in sorting method
for (int i = 0; i < 4; i++)
System.out.println(people[ i
]);
}
} |
Output:
7
11
12
18
20
Felix
George
Henry
Wally |
|
Notice that this built-in routine (shown above) sorts into "ascending" order only.
|
• binarySearch(array, value) - returns a -1 if the key "value" is not found in the array. The array must first be sorted by the Arrays.sort method. |
Example - Using Arrays.binarySearch( )
//
short demo to show built-in searching routine at work
import java.util.Arrays; //notice the new required import statement
public class SearchTest
{
public static void main(String[] args)
{
String[ ] people = "Wally","Henry","Felix","George"};
Arrays.sort(people); //use
built-in sorting method
for (int i = 0; i < 4; i++)
System.out.println(people[ i
]);
//Searching for location of "George" in sorted array
int index = Arrays.binarySearch(people,"George");
System.out.println("George located at index "+index);
}
} |
Output:
Felix
George
Henry
Wally
George located at index 1
|
|
|

Return to Unit Menu | JavaBitsNotebook.com | MathBits.com | Terms
of Use | JavaMathBits.com
|