|
Creating A Sequential Access File
(Writing TO a file!)
When creating a file, we are dealing with OUTPUT - information traveling "out" of our program, through the stream buffer, to a separate text file which will reside in Eclipse in a location such as "src/NewFile.txt".
Keep in mind that text files can also be written and viewed using a text editor.
|
A text editor can be used to view, or create, a file. Be careful of spacing.
If using MSWord to create the file, be sure to save it as a plain text file. |
|
When creating files, we will be using the class PrintWriter which prints formatted representations of objects to a text-output stream. It establishes the stream.

PrintWriter sends values of any type to a text file (by way of a stream) using print() and println() methods. Requires: import java.io.*;
When creating a new file, if a file of the same name already exists, the pre-existing file will be erased and replaced with the new file. |
It will be necessary to utilize try ... catch to prevent a syntax error,
since PrintWriter throws a FileNotFoundException.
Be sure to close the stream when the program is finished sending data.
This will complete the file, making it available for use by your program or other programs.
out.close();
print(String value): a method of PrintWriter which sends the characters from the String to the output stream. No "new line" character is sent.
println(String value): a method of PrintWriter which sends the characters from the String to the output stream, followed by "new line" separator.
When creating a file, nothing will appear in the console window. To see if your file was successfully created, you must view the text file itself.
You can code a "finished" message to the console to let you know the file was created, but you will not actually "see" the file. |

Steps to create (or write to) a sequential access file: |
1. Create a handle to the file by passing the name of the file to a File object:
File nf = new File("src/NewFile.txt");
2. Establish the stream that PrintWriter will use:
PrintWriter pw;
3. Establish a try ... catch to deal with possible exceptions.
If a program dealing with file access is not working properly, it is often hard to determine if the problem is within the program or if the problem is within the file access.
4. Attach the stream to the file:
pw = new PrintWriter(nf);
5. Write data to the file through the stream:
pw.println("Ed, Carol, John, Sue");
The data must be separated with space characters (white space, commas) or end-of-line characters (carriage return), or the data will run together in the file and be unreadable. Try to save the data to the file in the same manner that you would display it on the screen.
6. Close the file:
pw.close();
Closing the file writes any data remaining in the stream
buffer to the file, releases the file from the program, and updates the file directory to reflect the file's new size. As soon as your program is finished accessing the file, the file should be closed. Most systems close any data files when a program terminates. Should data remain in the buffer when the program terminates, you may loose that data. Don't take the chance -- close the file!
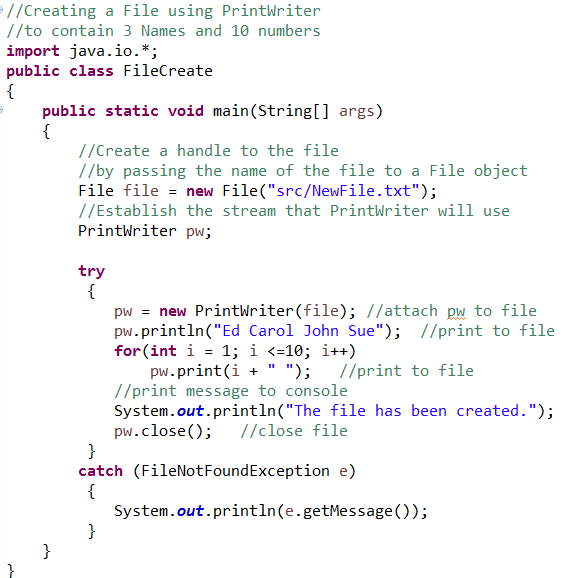
The file
NewFile.txt will be created:
Ed Carol John Sue
1 2 3 4 5 6 7 8 9 10 |

Return to Unit Menu | JavaBitsNotebook.com | MathBits.com | Terms
of Use | JavaMathBits.com
Notice: These materials are free for your on-line use at this site, but are not free for the taking.
Please do not copy these
materials or re-post them to the Internet, as it is copyright infringement.
If you wish hard-copies of these materials, refer to our subscription area, JavaMathBits.com.
Help us keep these resources free. Thank you. |
|