Detecting Errors and Exception Handlers
We have seen a multitude of error messages (everyone makes programming mistakes) and we have devised error trapping procedures to prevent users from sabotaging (crashing) a program by entering the wrong input. It's time to expand our error trapping skills.
An exception is an error that affects program execution. If an exception is not handled (dealt with), the program terminates. You most likely have seen the term "exception" in your past programming endeavors. If you asked a user to enter an integer (that would be stored in an int), and the user entered 2.4, the message "Exception in thread "main" java.util.InputMismatchException" appeared in red in the console as the program stopped execution.
In certain cases, an "exception handler" may be used to provide the user with an error message as to what went wrong, before the program abruptly ends. It may also enable the program to deal with exceptional situations and continue its normal execution.
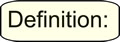 |
An exception handler is a block of code that performs an action when an exception occurs. |
|
You have already been dealing with potential errors when you "error trapped" your programs to guarantee that your user-input complied with your directions. Consider this simple program to demonstrate integer division, where the problem of division by zero could crash the program with the error message Exception in thread "main" java.lang.ArithmeticException: / by zero at Errors.main(Errors.java:11):
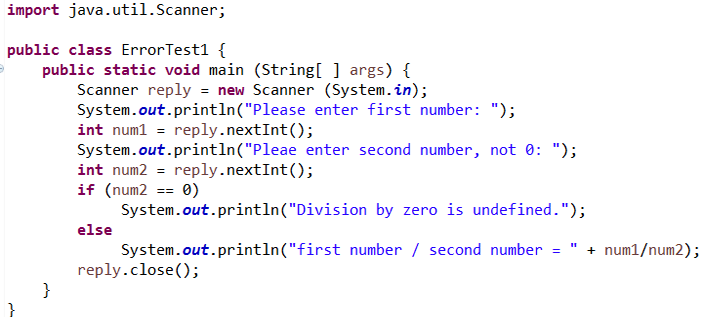
By including the if/else error trapping statements, the programmer has dealt with the situation of the user mistakenly entering the second number as zero. Instead of the program crashing, the program simply prints a message regarding division by zero (no crash). Problem avoided!
Exception handling is a more sophisticated process of dealing with potential errors in more complex programs. It is program code that deals with the anticipated exception and allows the program to continue after dealing with the exception. Let's look at a more sophisticated version of error trapping our division by zero problem.
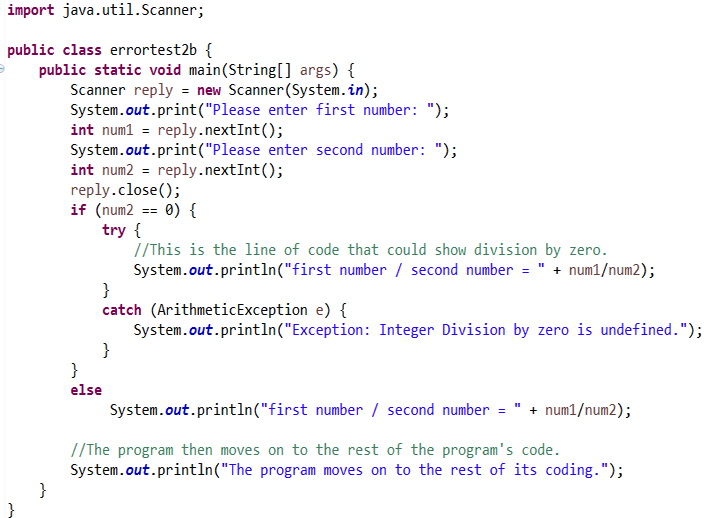
|