Using A Sequential Access File
(Reading FROM a file!)
Before you attempt to read from a sequential file, you will need to know the general format of the file and the type of data needed to receive the input. This can be accomplished by scanning the file in text format prior to opening the file for input. Know your file!
|
When reading from a file, we will be using the class Scanner.
Requires: import java.util.Scanner;
This is the same Scanner class that we used with the standard input stream.
Scanner reply = new Scanner(System.in);
When working with files, the standard input stream is connected
to the file by a Scanner object.
File newfile = new File("YourFile.txt"); // creates a File object
Scanner reply = new Scanner(newfile); // connect a Scanner to the file
Once established, the same methods that we use with familiarity from Scanner (such as nextInt()) are still available for our use.
reply.nextInt(); - returns an integer.
All white space is overlooked (skipped), including empty spaces, end-of-line character, and tab) that are located before the integer.
reply.nextDouble(); - returns a double.
reply.next(); - returns the next String value made up of characters up to, but not including, the next white space, end-of-line character, or other specified delimiter. Think of reading one word (without white space in the word).
reply.nextLine(); - returns the String value made up of all characters up to, but not including the end of the current line.
Remember the problems encountered with the Scanner class when accessing a String variable after accessing an int variable in the same stream. The String will pick up the left over newline character from the int, instead of picking up the next string item.
One way to deal with this problem is to include an additional reply.nextLine(); below each input of double or int data to "eat up" the remaining newline character which is causing the problem.
|
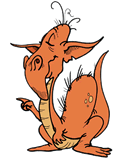 |
|

When working with files, we will now need some additional ammunition when reading a file, to determine if the input stream contains unread data.
reply.hasNext(); - returns true if the scanner (stream) has another token in its input. The scanner will not advance past any input. (The term "token" refers to Java elements such as keywords, variables, constants, special characters, operations, etc.)
reply.hasNextInt(); - returns true if the next set of characters in the input stream (scanner) can be read in as an int. If they cannot be read as an int or the end of the file is reached, a false is returned.
reply.hasNextLine(); - returns true if there is another line in the input stream.
reply.hasNextDouble(); - returns true if the next token in the scanner's input stream can be read as a double value.

When reading data from a sequential file, you MUST be aware of the necessary variable types needed to access the information in the file.
When working with an unknown file:
A text editor can be used to view the contents of a file. This allows you to become familiar with the variable status of an unknown file. |
Files may contain a great deal of data which are usually dealt with using a loop of some sort. A command such as while(reply.hasNextInt()) will be true as long as the next set of characters in the input stream can be read as an int. If they cannot be read as an int or the end of the file has been reached, a false is returned and this looping process will end.
|