|
We will be using Swing for all of our graphics applications. Remember that we are using JPanel as our drawing surface. A JPanel is a portion of a JFrame.
We will be discussing frames in more detail in the next unit. |
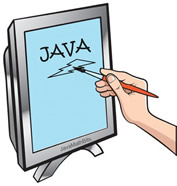 |
Coordinate Axes:
When working with graphics, a coordinate system is
essential for locating objects. You probably
remember your mathematical coordinate axes system which
utilizes an x-axis and a y-axis. Unfortunately, the
axes for Java graphics are somewhat different.
|
(0,0) Window |
The coordinate system for the
Java graphing
window positions (0,0) in the
upper left hand corner. The grid is then numbered in a
positive direction on the x-axis (horizontally to the right) and in
a positive direction on the y-axis (vertically going down).
|
|
Basic Methods from the Graphics class:
Line |
drawLine(int x1, int y1, int x2, int
y2);
Used to draw a straight line from point (x1,y1) to
point (x2,y2).
g.drawLine(35, 45, 75, 95);
To draw a POINT, use the drawLine method and supply the same one point for both arguments of the method.
|
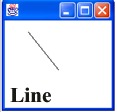 |
Rectangle |
drawRect(int x, int y, int width, int
length);
g.drawRect(35, 45, 25, 35);
Used to draw a rectangle with the upper left
corner at (x,y) and with the specified width and length. |
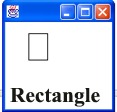 |
Round Edge Rectangle |
drawRoundRect(int x, int y, int width, int
length, int
arcWidth, int arcHeight);
g.drawRoundRect(35,45,25,35,10,10);
Used to draw a rounded edged rectangle. The
amount of rounding is
controlled by arcWidth and arcHeight. |
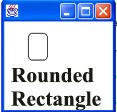 |
Oval / Circle |
drawOval(int x, int y, int width, int length);
g.drawOval(25, 35, 25, 35);
g.drawOval(25, 35, 25, 25); → circle
Used to draw an oval inside an imaginary rectangle whose
upper left corner is at (x,y). To draw a circle keep the
width and length the same. |
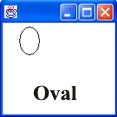 |
Arc |
drawArc(int x, int y, int width, int length,
int startAngle, int arcAngle);
g.drawArc(35,
45,
75, 95, 0, 90);
Used to draw an arc inside an imaginary rectangle whose
upper left corner is at (x,y). The arc is drawn from the startAngle
to startAngle + arcAngle and is measured in degrees. A startAngle of 0º points horizontally to the right
(like the unit circle in math). Positive is a counterclockwise rotation
starting at 0º. |
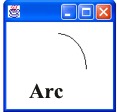 |
Polygon |
drawPolygon(int x[ ], int y[ ], int n);
int [ ] x = {20,
35, 50, 65, 80, 95};
int [ ] y = {60, 105, 105, 110, 95, 95};
g.drawPolygon(x, y, 6);
Used to draw a polygon created by n line segments. The command will close the polygon.
(x-coordinates go in one array with accompanying y-coordinates
in the other) |
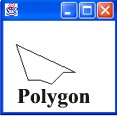 |
String
(text) |
drawString(String str, int x, int y);
g.drawString("Java is cool!", 40, 70);
Draws a string starting at the point indicated by (x,y).
Be sure you leave enough room from the top of the screen for the
size of the font. |
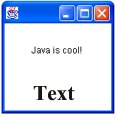 |
Code to produce a drawing program: |
When working with graphics, we will need to import:
import java.awt.*;
import javax.swing.*;
The javax prefix is used to signify a package of Java extensions.
All of our drawings will occur in a JPanel.
A JPanel draws its content in the method public void paintComponent (Graphics g);
Each of the drawing components (such as those shown above) is capable of drawing itself, which will be done automatically. You will not be "calling" this method. This method will be called by the system.
In addition, we will include a call to super.paintComponent(g); which will prevent problems with drawing colors in relation to the background color.
//Method format for the drawing method:
public class DisplayGraphics extends JPanel
{
public void paintComponent (Graphics g)
{
super.paint(Component(g);
// the drawings occur here
}
Now, in main:
public static void main(String[ ] args)
{
//Establish the frame
DisplayGraphics d = new DisplayGraphics( );
JFrame f = new JFrame("Title of the frame");
f.add(d); //adds DisplayGraphics to the frame for viewing
f.setSize(400,400); //sets the coordinate size of frame
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); //closes frame
f.setLocationRelativeTo(null); //centers the frame on screen
f.setVisible(true); //makes frame appear on screen
}
}
Note: The code above shows the frame's title being set in the line
JFrame f = new JFrame("Title of the frame");
It is also possible to separate the title to its own coding line, such as
JFrame f = new JFrame( );
f.setTitle("Title of the frame"); |

// Example of DRAWING
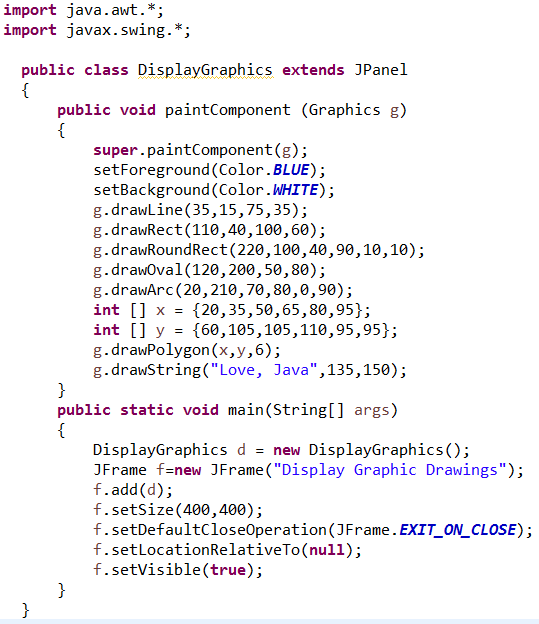
Display:
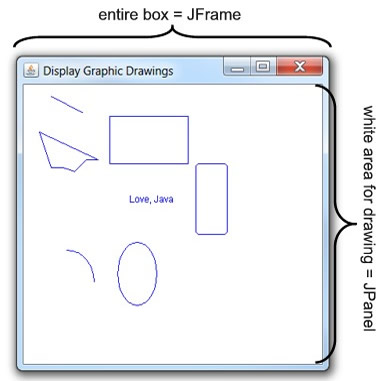
Comments:
You will notice that this example used
import java.awt.*;
import javax.swing.*;
While this general importing (*) of the entire package is not a problem in this program, it is considered more beneficial to import the specific methods needed for the program to save a bit of memory and avoid potential "overlap" problems (such as the Time method existing in more than one package).
import java.awt.Color;
import java.awt.Graphics;
import javax.swing.JFrame;
import javax.swing.JPanel;
FYI: The third line of this program gives a "Warning" referencing "serial UID".
The purpose of the serial UID is to forbid serialization and deserialization of the same classes in different versions, especially when there is not forward compatibility (eg. new version of the class cannot be serialized/unserialized by a previous definition).
This circumstance occurs rarely and is usually associated with projects by different authors that are interacting with one another. You can ignore this warning.
|
I have no errors, but I cannot see my window frame when I hit run.
It may be the case that a console (a frame), which you think is closed, is still running behind the scene. You may not be able to "see" this "running" console but pausing over the RUN button will show a statement about a running console existing.
Look for the grey double-X on the console display (on the right-hand side) to remove inactive consoles, and see if your red TERMINATE box shows up again. Continue until the red button does not return.
The drop-down arrow (circled in red) will show you if windows are still up and running (in the background).
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Using this code line should prevent a pile-up of running graphic windows.
If you should, by accident, close the CONSOLE tab, you can reinstate it by going to the menu bar and choosing, Window → Show View → Console. |

Return to Unit Menu | JavaBitsNotebook.com | MathBits.com | Terms
of Use | JavaMathBits.com
Notice: These materials are free for your on-line use at this site, but are not free for the taking.
Please do not copy these
materials or re-post them to the Internet, as it is copyright infringement.
If you wish hard-copies of these materials, refer to our subscription area, JavaMathBits.com.
Help us keep these resources free. Thank you. |
|