So, how do we incorporate graphics into our java code?
At this point in Unit 9, the following format will get you started drawing.
You will fill in the red areas with your programming code.
The other items should remain constant, for now.
import java.awt.*;
import javax.swing.*;
public class graphicsName extends JPanel
{
public void paintComponent(Graphics g)
{
super.paintComponent(g);
g.setColor(Color.green);
int [ ] x = {75,
150, 120};
int [ ] y = {75,
140, 50};
g.fillPolygon(x, y,
3);
}
public static void main (String[ ] args)
{
graphicsName d = new graphicsName();
JFrame f = new JFrame();
f.add(d);
f.setSize (300,300);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setLocationRelativeTo(null);
f.setVisible(true);
}
}
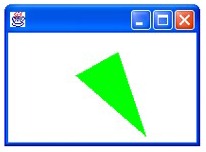 |