import java.awt.*;
import javax.swing.*;
public class Demo1 extends JPanel
{
public void paintComponent(Graphics g)
{
super.paintComponent(g);
setBackground(Color.WHITE);
int x = 50, y = 50, width = 40, height = 40;
g.setColor(Color.red);
for (int i = 1; i <= 7; i++)
{
g.drawOval(x, y, height, width);
width = (int) (width*1.3);
height = (int) (height*1.3);
}
}
public static void main(String[] args) {
Demo1 p = new Demo1();
JFrame f = new JFrame();
f.setSize(300, 300);
f.setDefaultCloseOperation
(JFrame.EXIT_ON_CLOSE);
f.add(p);
f.setVisible(true);
}
}
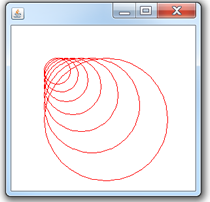
|
import java.awt.*;
import javax.swing.*;
public class Demo2 extends JPanel
{
public void paintComponent(Graphics g)
{
super.paintComponent(g);
setBackground(Color.WHITE);
int x = 50, y = 50, width = 40, height = 40;
g.setColor(Color.red);
for (int i = 1; i <= 4; i++)
{
g.drawRect(x, y, height, width);
x = x + 10; y = y + 10;
width = (int) (width*1.3);
height = (int) (height*1.3);
}
}
public static void main (String[] args)
{
Demo2 p = new Demo2();
JFrame frm = new JFrame();
frm.setSize (300,300);
frm.setDefaultCloseOperation
(JFrame.EXIT_ON_CLOSE);
frm.add(p);
frm.setVisible(true);
}
}
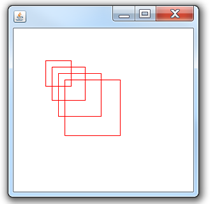
|
import java.awt.*;
import javax.swing.*;
public class Demo3 extends JPanel
{
public void paintComponent(Graphics g)
{
super.paintComponent(g);
setBackground(Color.WHITE);
int x = 120, y = 80, width = 140, height = 140;
g.setColor(Color.red);
for (int i = 1; i <= 6; i++)
{
g.drawOval(x, y, height, width);
x = x - 20;
y = y + 20;
width = (int) (width - 20);
height = (int) (height - 20);
}
}
public static void main (String[] args)
{
Demo3 p = new Demo3();
JFrame frm = new JFrame();
frm.setSize (300,300);
frm.setDefaultCloseOperation
(JFrame.EXIT_ON_CLOSE);
frm.add(p);
frm.setVisible(true);
}
} 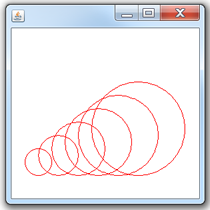
|
import java.awt.*;
import javax.swing.*;
public class Demo4 extends JPanel
{
public void paintComponent(Graphics g)
{
super.paintComponent(g);
setBackground(Color.WHITE);
int x = 150, y = 150, width = 140, height = 140;
g.setColor(Color.red);
for (int i = 1; i <= 6; i++)
{
g.drawArc(x, y, height, width, 0, 90);
x = x - 20;
y = y + 20;
width = (int) (width - 20);
height = (int) (height - 20);
}
g.drawLine(220,150, 20,285);
g.drawLine(290,220, 20,285);
}
public static void main (String[] args)
{
Demo4 p = new Demo4();
JFrame frm = new JFrame();
frm.setSize (320,350);
frm.setDefaultCloseOperation
(JFrame.EXIT_ON_CLOSE);
frm.add(p);
frm.setVisible(true);
}
}
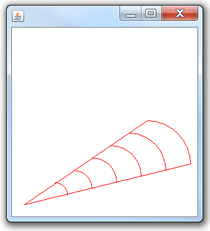
|