Time and Date Methods
The information on this page is for Java 8 or later only. |
In the past, "time" could be accessed in Java using methods from the Date class and the Calendar class, which proved to be somewhat cumbersome and non-intuitive in design.
Thankfully, Java SE 8 has developed a new date and time library (package)
called java.time.
The important classes included in the java.time package are:
• LocalDate - deals with date only
• LocalTime - deals with time only
• LocalDateTime- deals with both data and time
Both LocalDate and LocalTime use the system clock in the time zone of the system running the application.
|
To obtain specific info: |
.getYear();
|
.getMonth(); |
.getDayOfMonth(); |
|
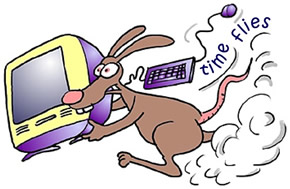 |
Remember to import java.time.*; |
LocalDate will produce a year-month-day date without time.
Example:
LocalDate today = LocalDate.now();
System.out.println("Today's date: " + today);
Output: Today's local date: 2016-10-26 //notice the style of the printing
Example:
//Obtain only specific items in the date
LocalDate today = LocalDate.now();
int year = today.getYear();
int month = today.getMonthValue();
int day = today.getDayOfMonth();
System.out.format("Year : %d Month : %d day : %d \n", year, month, day);
Output: Year : 2014 Month : 1 day : 14

LocalTime
will produce a time of day.
Example:
LocalTime time = LocalTime.now();
System.out.println("local time now: "+ time); |
Hours, Minutes, Seconds, Nanoseconds
1 hour = 60 minutes
1 minute = 60 seconds
1 second = 1000000000
nanoseconds
1 second
= 1 x 109 nanoseconds |
|
Output:
local time now: 16:33:33.369
Printed in hours, minutes, seconds, nanoseconds.
Example:
//Obtain only specific items in the time
LocalTime time = LocalTime.now();
int hour = time.getHour();
int min = time.getMinute();
int sec = time.getSecond();
System.out.format("Hour : %d Minute : %d Second : %d \n", hour, min sec);
Output: Hour: 13 Minute : 32 Second: 43

LocalDateTime
will produce date and time.
Example:
LocalDateTime datetime = LocalDateTime.now();
System.out.println("local date and time now: + datetime);
Output:
local date and time now: 2016-10-30T10:56:47.023
Notice the "T" used to separate the date from the time

Due to the manner in which LocalTime represents time, finding the amount of elapsed time can be quite involved. There is an easy way to estimate elapsed time by using System.currentTimeMillis();
This method returns the current time (long integer) in milliseconds, which is the difference between the current time and midnight, January 1, 1970 (Universal Time).
Example:
long startTime = System.currentTimeMillis();
. . . more code here
long endTime = System.currentTimeMillis() - startTime;
long seconds = endTime/1000; //convert milliseconds to seconds

The computer can print information very, very quickly to the screen. It may be necessary to slow down how quickly text is appearing so the user can digest what is happening before given more information. At times, you may even want to pause the display, if only for a few seconds.
//Pause the screen for 1 second (1000 milliseconds = 1 second)
try
{
Thread.sleep(1000);
}
catch(InterruptedException ex)
{
Thread.currentThread().interrupt();
}

//Press ENTER to continue option
System.out.println("Press ENTER to continue.");
Scanner pause = new Scanner(System.in);
pause.nextLine();