|
What are Methods?
A method is a
set of code which is referred to by name and can be called (invoked) at
any point in a program simply by utilizing the method's name.
Think of a method as a subprogram that acts on data and may, or may not, return a
value. |
Each method has
its own name. When that name is encountered in a program, the execution
of the program branches to the body of that method. When the method is
finished, execution returns to the area of the program code from which
it was called, and the program continues on to the next line of code.
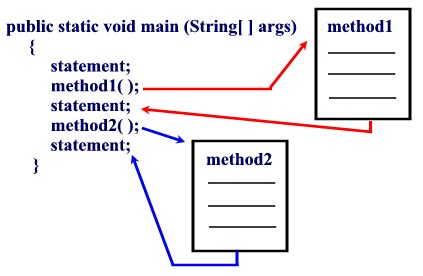
Good programmers write in a
modular fashion which allows for several programmers to work
independently on separate concepts which can be assembled at a later
date to create the entire project. The use of methods will be our
first step in the direction of modular, top-down programming.
Top-down programming is an approach to writing programs that breaks the task of the program down into smaller subtasks. These subtasks can be implemented using methods.
Methods are time savers, in that they allow for the repetition of
sections of code without retyping the code. In addition, methods
can be saved and utilized again and again in newly developed programs.

A method consists of a declaration and a body.
The method declaration includes the access level, return type, name, and possible parameters. The method body contains the statements implemented by the method.
Method Declaration: access level, return type, name, parameters
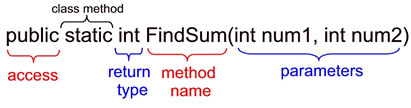
This declaration shows a public access level implying other classes can call this method. The term static declares this method as a class method implying it can be called from the class itself. The int return type implies that an integer value will be returned by this method. The name of this method is FindSum. This method will receive two integers (parameters) to be used within the computations perforemd by this method.
|
Method Declaration + Body:
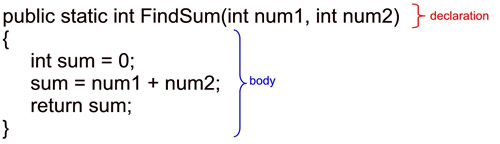
In Java, the term "declaration" often refers to the declaration along with the body of a method. You may also see the declaration along with the body referred to as "defining" the method, or the method definition. Unlike C/C++, Java does not emphasize a distinction between the terms "declaration" and "definition" as related to methods.
|

You have already been using methods when you use
System.out.print( ) and System.out.println( ).
(These are referred to as build-in methods.)
There are two
basic types of methods:
Built-in: Build-in
methods are part of the compiler package, such as System.out.println( ) and System.exit(0). |
User-defined: User-defined
methods are created by you, the programmer. These methods take-on
names that you assign to them and perform tasks that you create. |

How to invoke (call ) a method (method
invocation):
When a method is
invoked (called), a request is made to perform some action, such as
setting a value, printing statements, finding a sum, returning an answer, etc.
The code to invoke the method contains the name of the method to be
executed and any needed data that the receiving method requires (parameters).
The required data for a method are specified in the method's parameter
list.
 Consider this built-in method that we have used to obtain user input:
int
number = reply.nextInt(); //returns an integer value from the user
The method name is "nextInt"
which is defined in the class "Scanner".
Since the method is defined in the class Scanner, the Scanner variable "reply" becomes the calling object. This particular method returns an integer
value which is assigned to an integer variable named number. |
You invoke (call) a
built-in method by writing down the calling object followed by a dot, then the name of
the method, and finally a set of parentheses that may (or may not) have
information (called parameters) for the method. |
 When writing a method (user-defined), you will be responsible for creating the actual coding of the method. Consider this method that we named FindSum which will receive two numbers (integers) and return their integer sum.
public static int FindSum (int num1, int num2) {
The method name is "FindSum"
which will be defined by code written by the programmer. This particular method returns an integer
value which will need to be stored in some integer location. And this method needs to receive two integer values to be used to produce the sum. (We saw the body of this method in the statements above.)
When you name a method, verbs are a good choice since they indicate an action, which will remind you of what the method will be used to accomplish.
|
*** IMPORTANT NOTE:
Any variables declared within a method are referred to as being of local scope.
These local variables are "known" only to that method and will not be "known" when you return to main, or go to another method. |

FYI: It is possible to code two or more methods with the same name, but with unique combinations of parameters. This process is known as overloading. In such a case, the number of parameters and/or the data types of the parameters, form a designation of the method, where each method's designation must be unique. We will not be addressing overloading of methods at this level.

For those of you who are familiar with other programming languages,
the Java "method" is similar in concept
to what C and C++ call "functions"
and QBasic and QuickBasic call "subroutines".
Pascal uses the terms "subroutines, functions and procedures".

Return to Unit Menu | JavaBitsNotebook.com | MathBits.com | Terms
of Use | JavaMathBits.com
|