Return to Unit Menu |
JavaBitsNotebook.com |
MathBits.com |
Terms
of Use |
JavaMathBits.com
Introduction to a Graphical User Interface
In today's world, it is assumed that users will be able to interact with computer applications. Everyone understands how to click buttons and check boxes, for example.
In the Graphics unit, we saw the development of a working "window" and the capability of drawing graphics, including mouse-action. This unit will concentrate on how a user can interact with that "window".
A GUI (Graphical User Interface pronounced "gooey") program is an "event-driven" program. Those button clicks and checked boxes from the user's keyboard generate "events". A GUI program must then be able to respond as those events occur. This will be accomplished by utilizing interfaces and classes from the Java AWT (Abstract Window Toolkit) stored in the java.awt package.
We saw, in the Graphics unit, the use of Swing to display a frame which acts as the display window for an application. Components are then added to the frame and events (such as clicking the mouse) are "handled" as the user interacts with the components. This handling of events is accomplished by coding an event listener that "listens" for the event to occur. Read more about event listeners under JButtons. |

|
You will need to remember the basic window formatting from the Graphics unit. The following code creates a basic window (frame). |
|
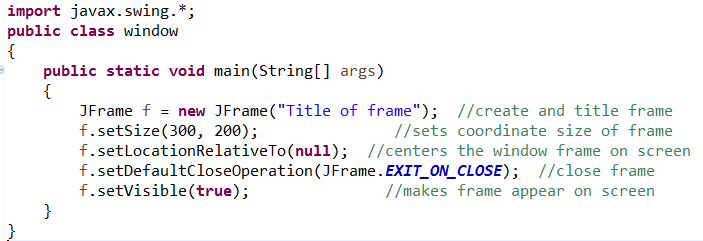
Remember, if you do not include the line setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
when the user clicks the close button, the frame closes, but the application continues running. The close button only closes the frame by default, but doesn't end the application. |

We have also seen the use (in the past) of the "container" JPanel within the JFrame.
While it is possible to "nest" JPanels, we will be concentrating on the use of one JPanel per frame. We will also be concentrating on adding "components" to our JPanel in the form of buttons, check boxes, radio buttons, etc.
|
Methods that pertain to the JPanel: |
add(component)
setLayout(layout)
Note: JPanel is the default container for JFrame.
|

Content Pane:
In Java Swing, the container layer which is used to hold components is referred to as the "content pane." The default container is the JPanel.
For GUI programs, it is necessary to tell the program that you will be using the JPanel as your content pane. To set the contents of the content pane, we will be using the method JFrame.add.
Note: older versions of Java used JFrame.getContentPane().add(), which has now been condensed to simply JFrame.add
JFrame frm = new JFrame(); //create & title frame
JPanel panel = new JPanel(); //create JPanel
frm.add(panel); //establish JPanel as container

The possibilities of working with GUIs is vast. Our work in this unit will only be an introduction to the world of GUIs. We will be taking a basic look at a select number of Swing components, layout managers, and event handlers to create applications with a graphical user interface.